Sending SMS notifications for WooCommerce orders is an effective way to keep store admins updated about new sales in real-time. ClickSend SMS is a reliable platform that integrates well with WooCommerce and allows you to send notifications seamlessly.
In this step-by-step guide, we’ll explore how to integrate ClickSend SMS into WooCommerce for sending SMS notifications to the admin when a new order is received.
Table of Contents
- Why Send SMS Notifications for WooCommerce Orders?
- Prerequisites for Integration
- Setting Up the ClickSend API
- The Complete Code Integration
- Step-by-Step Breakdown of the Code
- Testing the Integration
- Troubleshooting Common Issues
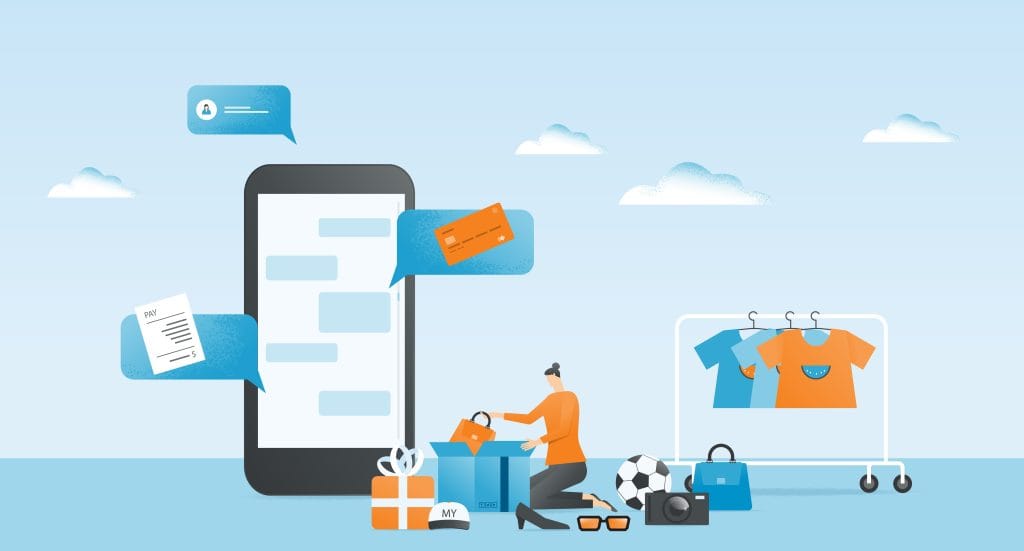
Why Send SMS Notifications for WooCommerce Orders?
SMS notifications are quick, effective, and provide instant updates that can be critical for running an eCommerce store. Sending SMS order notifications allows you to:
- React Quickly: Get notified immediately about new orders.
- Increase Efficiency: No need to continuously monitor your email inbox.
- Improve Workflow: Use real-time information to make operational decisions faster.
Prerequisites for Integration
Before integrating ClickSend SMS with WooCommerce, ensure that you have:
- WordPress Website with WooCommerce Installed: Ensure WooCommerce is properly configured to handle orders.
- ClickSend Account: Sign up for a ClickSend account to get your API credentials.
- ClickSend API Key: You need the API username and API key for authorization.
- Basic PHP Coding Skills: A basic understanding of PHP and WordPress hooks will be helpful.
Setting Up the ClickSend API
Step 1: Create an Account with ClickSend
First, create an account on ClickSend. Once logged in, you will need to access the API keys. Go to API Settings to find your username and API key.
Step 2: Install the ClickSend SDK
For this integration, we will be using the ClickSend PHP SDK. This library will help make the connection to ClickSend’s services. You can include the SDK via Composer or by downloading it directly.
To use Composer, run:
composer require clicksend/clicksend-php
Ensure that Composer is properly installed on your system before running this command.
The Complete Code Integration
Below is the code that you need to add to your theme’s functions.php file or as part of a custom plugin to integrate ClickSend SMS into WooCommerce for order notifications.
add_action( 'woocommerce_order_status_processing', 'synmek_call_when_processing' );
function synmek_call_when_processing( $order_id ) {
// Order object (optional but handy)
$order = new WC_Order( $order_id );
$order_name = $order->get_billing_first_name() . " " . $order->get_billing_last_name();
$customer_phone = $order->get_billing_phone();
$delivery_method = $order->get_shipping_method();
$order_date = $order->get_date_created();
$new_order_subject = "[Website Name] New Order Received on [" . $order_date . "]. Here is the order number: " . $order_id . " and for: " . $order_name . ". Here is their phone number: " . $customer_phone . " - Open the WOO app to view full order details";
// Configure HTTP basic authorization: BasicAuth
$config = ClickSend\Configuration::getDefaultConfiguration()
->setUsername('CLICKSEND USERNAME')
->setPassword('CLICKSEND API KEY');
$apiInstance = new ClickSend\Api\SMSApi(new GuzzleHttp\Client(), $config);
$msg = new \ClickSend\Model\SmsMessage();
$msg->setBody($new_order_subject);
$msg->setTo('YOUR PHONE NUMBER HERE');
$msg->setSource("sdk");
// \ClickSend\Model\SmsMessageCollection | SmsMessageCollection model
$sms_messages = new \ClickSend\Model\SmsMessageCollection();
$sms_messages->setMessages([$msg]);
try {
$result = $apiInstance->smsSendPost($sms_messages);
// Log success or further processing if needed
} catch (Exception $e) {
print_r($e);
}
}
Step-by-Step Breakdown of the Code
Step 1: Register a WooCommerce Action Hook
add_action( 'woocommerce_order_status_processing', 'synmek_call_when_processing' );
This line of code tells WooCommerce to call the synmek_call_when_processing
function when the order status changes to “processing”. The woocommerce_order_status_processing
hook is useful because it triggers only when the payment is successful and the order is being prepared.
Step 2: Define the Function
function synmek_call_when_processing( $order_id ) {
This function is where all the integration with ClickSend will take place. It receives the $order_id
parameter, which represents the ID of the order being processed.
Step 3: Retrieve Order Details
$order = new WC_Order( $order_id );
$order_name = $order->get_billing_first_name() . " " . $order->get_billing_last_name();
$customer_phone = $order->get_billing_phone();
$delivery_method = $order->get_shipping_method();
$order_date = $order->get_date_created();
These lines create an instance of the WC_Order
object and fetch important order details, including the customer’s name, phone number, and delivery method. This data will be used in the SMS notification.
Step 4: Construct the SMS Content
$new_order_subject = "[Website Name] New Order Received on [" . $order_date . "]. Here is the order number: " . $order_id . " and for: " . $order_name . ". Here is their phone number: " . $customer_phone . " - Open the WOO app to view full order details";
Here, we create the content of the SMS message. You can customize this message to include the details that are most relevant to you.
Step 5: Set Up ClickSend Authorization
$config = ClickSend\Configuration::getDefaultConfiguration()
->setUsername('CLICKSEND USERNAME')
->setPassword('CLICKSEND API KEY');
Here, you set up the ClickSend API authorization using the provided username and password (API key). Ensure that the credentials are correctly obtained from your ClickSend account.
Step 6: Create and Send the SMS
$apiInstance = new ClickSend\Api\SMSApi(new GuzzleHttp\Client(), $config);
$msg = new \ClickSend\Model\SmsMessage();
$msg->setBody($new_order_subject);
$msg->setTo('YOUR PHONE NUMBER HERE');
$msg->setSource("sdk");
$sms_messages = new \ClickSend\Model\SmsMessageCollection();
$sms_messages->setMessages([$msg]);
Here, we create an instance of the ClickSend SMS API and prepare the SMS message using the SmsMessage
model. Replace 'YOUR PHONE NUMBER HERE'
with the admin’s phone number where notifications should be sent.
Step 7: Sending the SMS and Handling Exceptions
try {
$result = $apiInstance->smsSendPost($sms_messages);
// Log success or further processing if needed
} catch (Exception $e) {
print_r($e);
}
This try...catch
block is used to send the SMS using the ClickSend API. If there’s an error during the API call, it will be caught and displayed for debugging purposes.
Testing the Integration
Step 1: Place a Test Order
To ensure everything works as expected, create a test order in WooCommerce. Set the order status to Processing and confirm that the SMS notification is sent to the configured phone number.
Step 2: Check for Errors
If the SMS is not received, check your error logs. Verify that the API credentials, phone number, and other details are correct. Ensure that the ClickSend SDK is installed properly and that there are no connectivity issues.
Troubleshooting Common Issues
- SMS Not Received:
- Double-check the ClickSend credentials (username and API key).
- Ensure that your phone number is correctly formatted (include the country code).
- Order Hook Not Triggering:
- Verify that the order status is set to Processing. The hook won’t trigger for orders with other statuses like “on-hold”.
- Composer Issues:
- If you face issues installing Composer, try using the ClickSend library directly by downloading it and including it manually.
Conclusion
Integrating ClickSend SMS with WooCommerce is a great way to enhance your store’s operational efficiency by keeping the admin up-to-date with new orders in real time. By following the steps outlined in this guide, you can ensure that you never miss an important order notification again. The integration is flexible and can be modified to suit specific business needs. If you need help with implementation or a custom WooCommerce solution feel free to get in touch with our expert WordPress development team.